July 8th, 2019
↳ Xamarin
How to Find the iOS Simulator's Database
When using CoreData in iOS, or manually using SQLite, sometimes it helps to be able to connect to your application's database with a browser, such as Navicat.
It can be tricky to find the file's location, since each iOS simulator, and each application installed to each simulator, have their own randomly generated UUID-named directory.
However, with a short bit of code, we can get the location of each app, at runtime.
If you already have an application, great! If not, you can use this Xamarin sample, which features CoreData.
To get started, let's add a simple logging method, and invoke it when the application launches.
Simply open the AppDelegate.cs
file, which should be located in the root of your iOS project, and add LogDocumentDirectory
.
Then, call it from FinishedLaunching
. You may need to implement this method, if it's not already stubbed out.
private void LogDocumentDirectory() { var documentUrls = NSFileManager.DefaultManager.GetUrls( NSSearchPathDirectory.DocumentDirectory, NSSearchPathDomain.User ); var documentDirectory = documentUrls.FirstOrDefault()?.ToString()?.Replace( "file://", string.Empty ); Console.WriteLine($"Document directory: {documentDirectory}"); } public override bool FinishedLaunching(UIApplication application, NSDictionary launchOptions) { LogDocumentDirectory(); // your existing code, possibly return true; }
When you run your app, you should see output similar to mine, with a different User directory (probably), and different
# line breaks added for legibility Document directory: /Users/josh/Library/Developer/CoreSimulator/Devices ⏎ /4A2368D0-398A-4C0C-975A-10F780D50930/data/Containers/Data ⏎ /Application/CB697916-52D3-4C0D-B787-D406121BC4B4/Documents/
To use that path via Finder, first open a window:
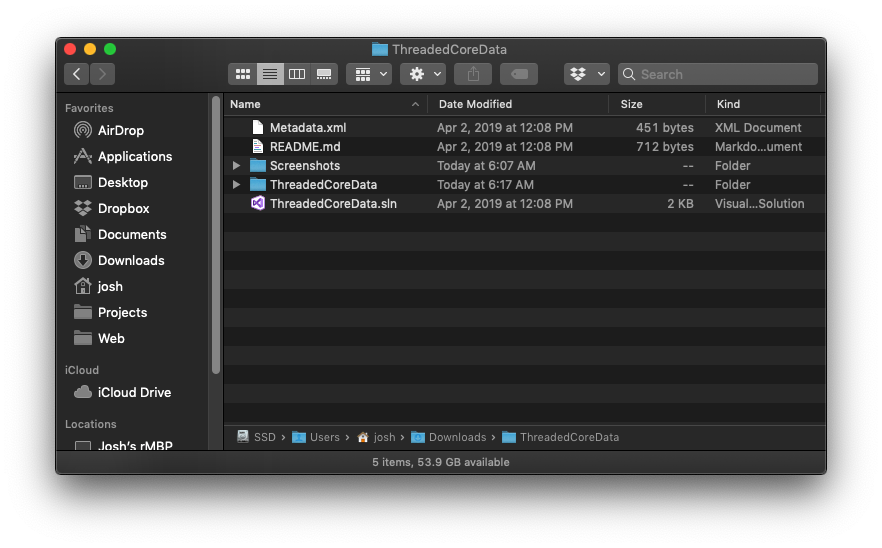
Then, open the "Go to Location" UI, with ⌘+⇧+G (Command+Shift+G):
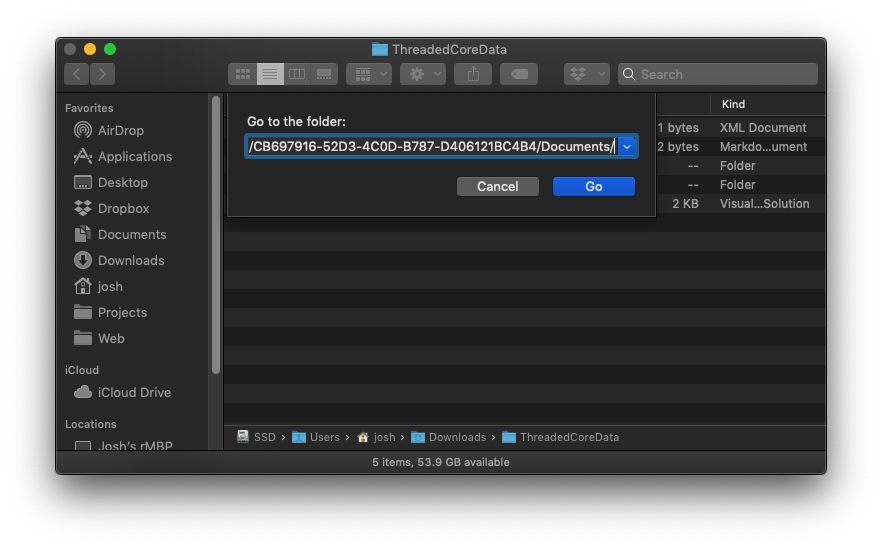
Paste in the path from the earlier Application Output, and choose "Go", and you should see your SQLite file:
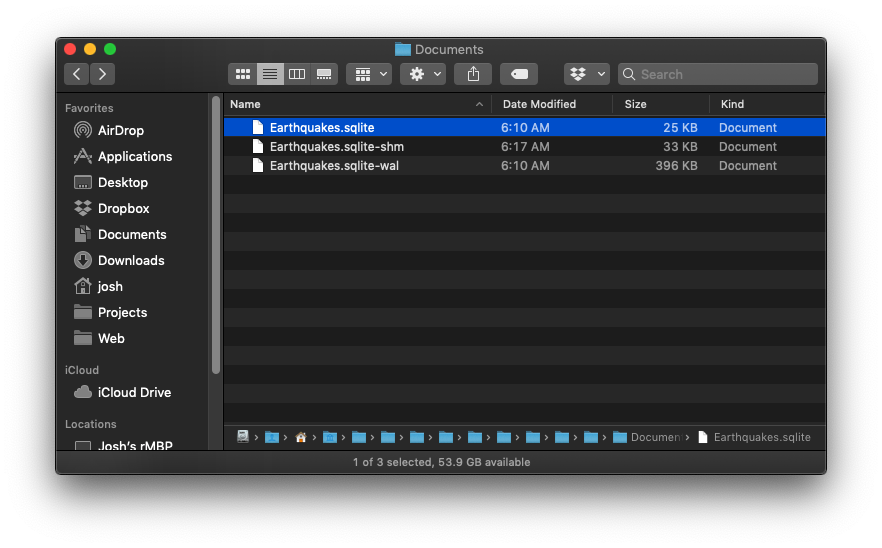
If you'd prefer to use the Terminal (or even better, iTerm2), just launch and cd
into it.
From there, you can use $ open .
to launch a Finder window, or use sqlite3
to query your database:
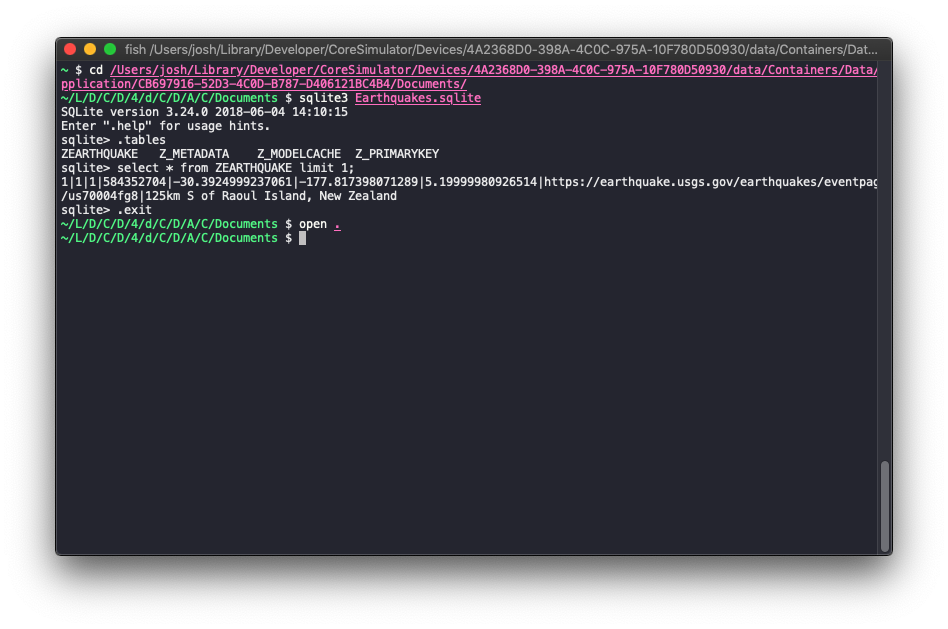
That's all it takes to find your app's database location.
Of course, this will work in Swift, if your application is being written natively!
Thanks for reading, I hope to see you again!
- Josh